Capstone Project: Week 9
- Paddy Benson
- May 11, 2024
- 4 min read
Updated: May 18, 2024
This week I wanted to focus on the game feel, more specifically the HUD elements for the player. Up to the this point all the information displayed was just in simple text elements. There was nothing wrong with it I just want my game to feel more special and unique. After brainstorming ideas on Photoshop I decided to go with a HUD that would hug the player on either side like this: ( ). I wanted one bar to be the speed of the player and the other for now is the lap count.
I decided to go about having a bar HUD through a radial fill, allowing me to set the fill amount within code and lerp between them. My first test used a full circle and so max speed was fill = 1, and min speed was 0.
// Set the fill amount of the speedometer bar
float minFillAmount = 0f;
float maxFillAmount = 1f;
float fillAmount = Mathf.Lerp(minFillAmount, maxFillAmount, mappedSpeed);
speedometerBar.fillAmount = fillAmount;
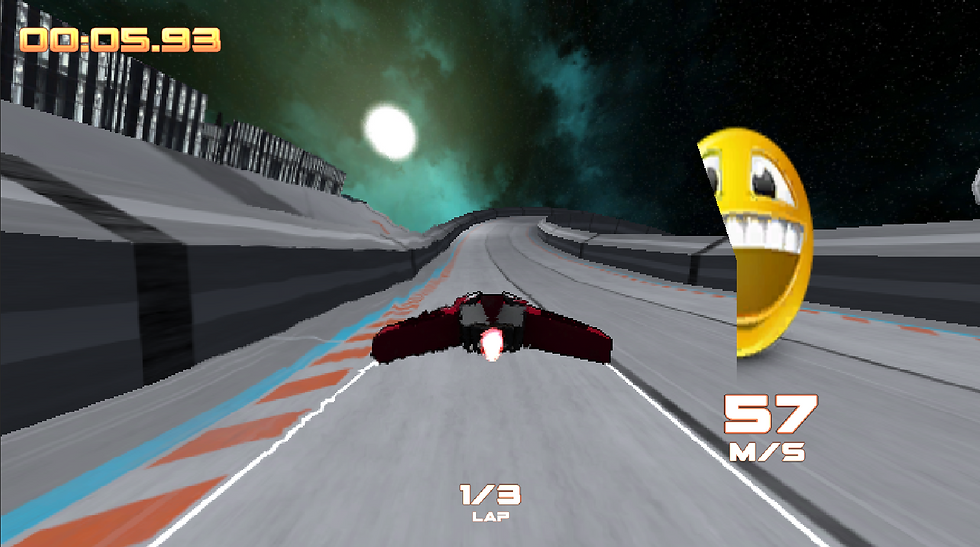
After seeing that it worked I then got about making the HUD two brackets instead of circles. This is as I want to siphon the players focus to the center of the screen and having the HUD elements border the player ship allows them to feel more immersed and in the cockpit. I made custom arc's in Photoshop but quickly ran into my first issue. The radial fills radius was different to that of the arc and so it wasn't following the path of the arc when moving. This led to the arc looking bad and uneven.
I solved this issue in a very simple and great fix. I made a single loop sprite in Photoshop:
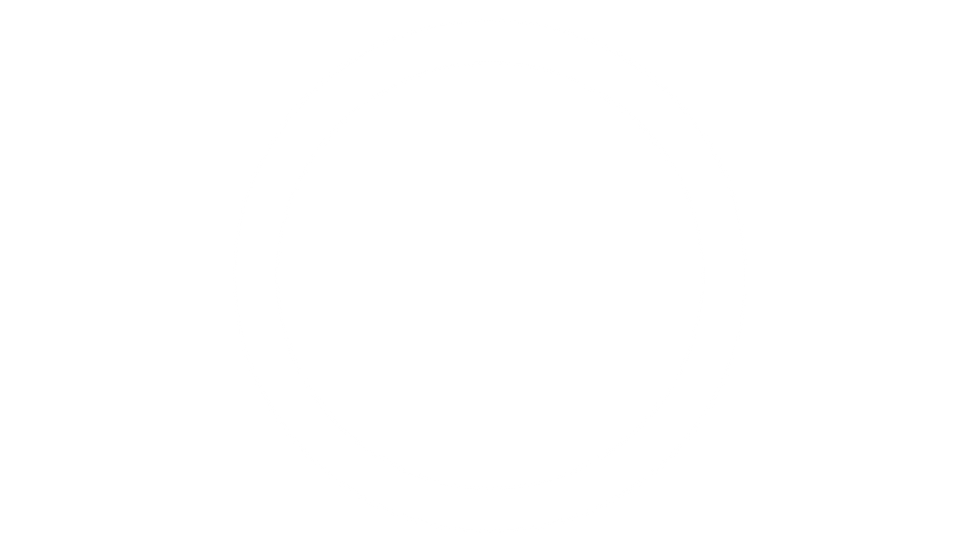
As I wanted more of a arc/bracket shape I simply divide the circle by four and set the max fill amount to be .25. The output of this worked great and as it was a true circle the radial fill worked perfectly.
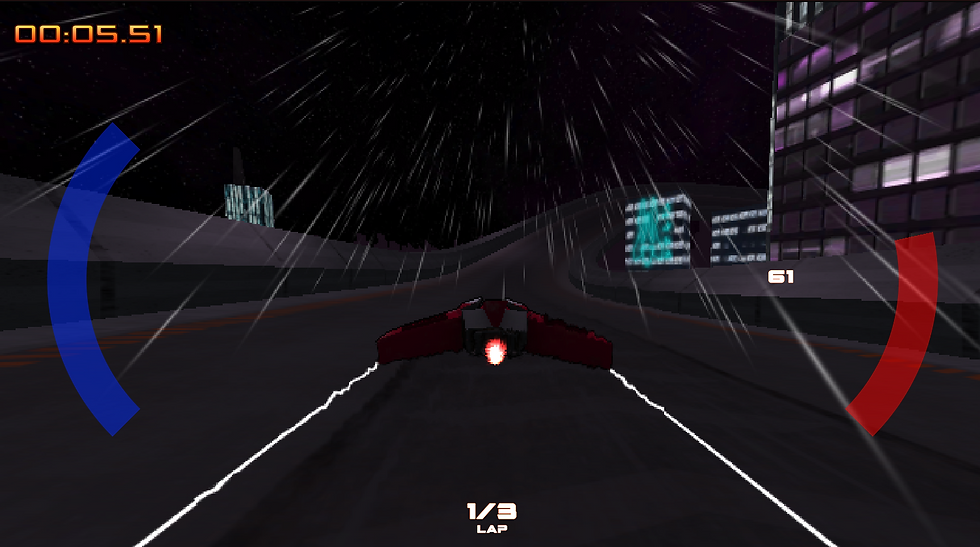
After getting the speed working I then worked on making a lap counter bar for the left side of the screen. This was more complicated for me and took a bit of fiddling to get right. At first I had it set to be more modular taking the lap count that could be changed and then dividing the arc by that amount to get each segment. However it never really worked properly, leading to the entire lap count system being somewhat broken. I'm not the best coder and so there would have been a solution however for my needs where the lap count will always be 3 anyway I took a more rudimentary, working, approach.
public void SetLapDisplay(int currentLap, int numberOfLaps)
{
currentLapText.text = currentLap + "/" + numberOfLaps;
// Calculate the fill amount for the lap bar
float fillAmount = (currentLap - 1) * 0.083f; // fill by 0.083 each lap
fillAmount = Mathf.Clamp(fillAmount, 0f, 0.25f); // Clamp the fill
// Set the fill amount for the lap bar
lapBar.fillAmount = fillAmount;
}
It woks by just dividing .25 by 3 (lap count) giving you .083 recurring. I then just add that on to the bar's fill each lap.
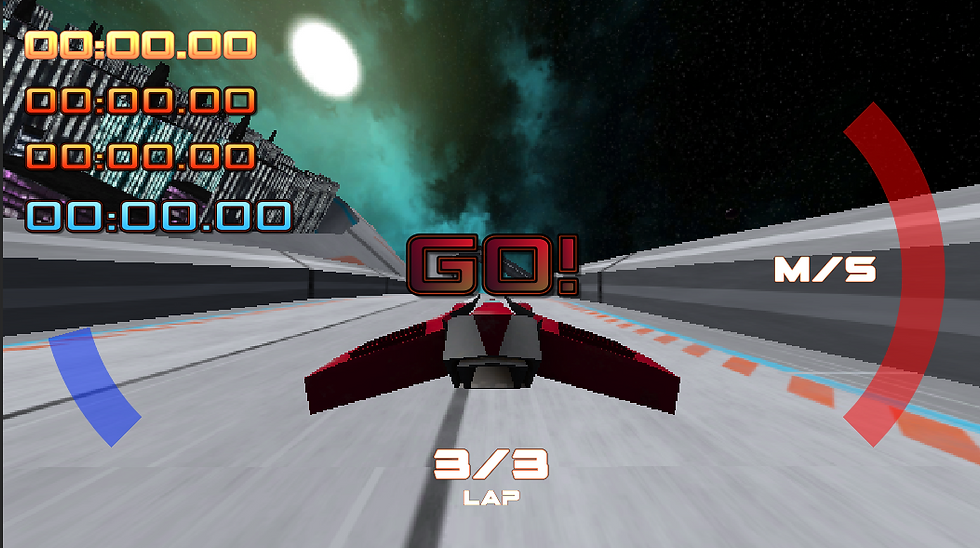
Seeing that it worked I then gave the illusion of segments by creating a sprite to cover the bar:
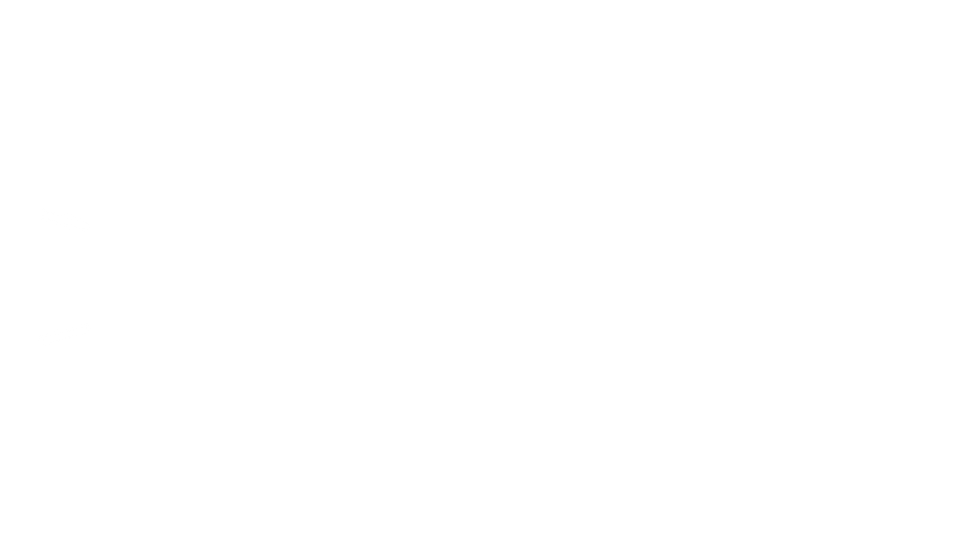
As well as background sprites to give the HUD a more put together look for now:
I was happy with it however it was missing an immersion that was needed and so I decided to go from a screen overlay to a world space HUD. By parenting it to the player character in game it made the game play feel much more alive and immersive as it moved with your ship.
This lead to me having to solve the issue of HUD clipping into walls and NPC's as it now inhabited world space. I did this by rendering the HUD over top of everything else so that no matter where it is it will always be displayed over top. This is done through a second camera with the exact same settings as the first and a culling mask on both. The main camera culls all UI while the second culls everything but UI.
After this I wanted to make the HUD even more emotive by adding a glow. My main goal currently is adding to the sense of both speed and urgency within game play and I think this definitely helped. By having two colours, one completely transparent for min speed and one bright red for max speed I can then simply lerp between them on the a glow sprite making it glow brighter the faster you are going.
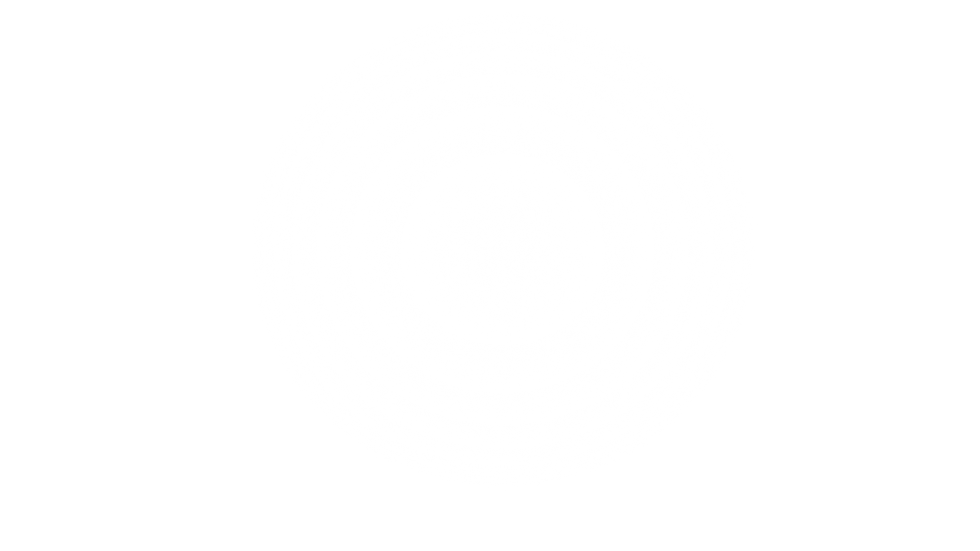
Color glowColor = Color.Lerp(minGlowColor, maxGlowColor, mappedSpeed);
// Set colour of glow
speedGlow.color = glowColor;
I then decided to do the same for the bar itself, but rather glow the red gets brighter the faster you are going:
Color speedColor = Color.Lerp(minSpeedColor, maxSpeedColor, mappedSpeed);
// Set colour of bar
speedometerBar.color = speedColor;
I have also been messing around with post processing and motion blur however haven't decided on a final implementation yet. A benefit of my approach is also as all sprites are white it allows for me to change the colour of them whenever I want in unity. That's all the HUD work I have done this week. I still need to do the timing and position but due to coding not being my main strength I am happy with my progress. As always here is a video to show it off:
留言