Capstone Project: Week 18
- Paddy Benson
- Aug 24, 2024
- 3 min read
This week I further worked on polishing the user feedback and overall feel of the game through both visual and audio cues.
I first worked on a control scheme layout image for the controls page. My original idea was to have images like this:
However once I placed them within my game due to the lower resolution they became extremely difficult to read and so I had to make the switch to a more simple grid layout. A bit of a downgrade aesthetically but it does it's intended job which is most important:
I then took a break from that to do bug fixing where I solved an issue that was exclusive to level 2 in which one of the AI would be registered multiple times when crossing the line causing them to place higher then they should. This was actual far simpler than I had worried and was just due to me having the wrong tag on certain game objects. The outcome of working while tired. I also added the cinematic camera for level 4 at the start of the race as well as the image and text for the level select screen.
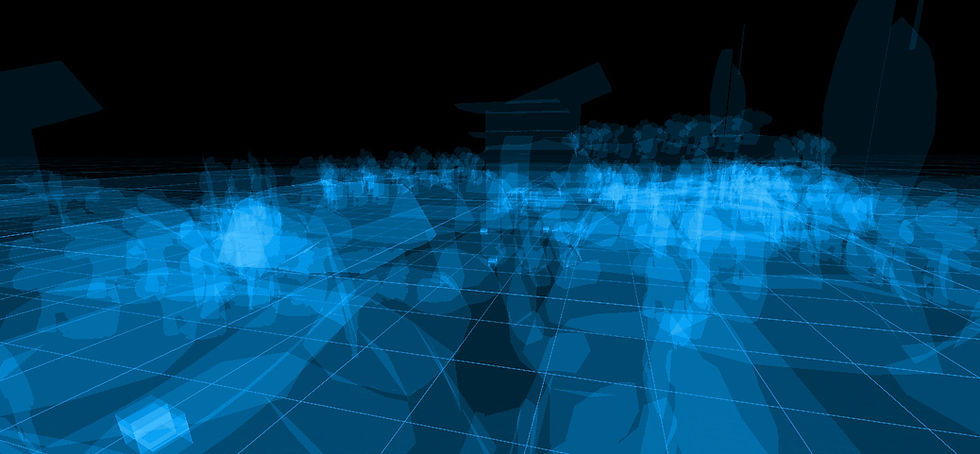
I also created both a logo and splash image for when my game is loaded up on a build.
I also added a warning inspired by Yakuza games telling the player to use a controller as it feels better:
I then added two more songs to my game to further boost the catalogue. You can find the full playlist here:
I then added more sound effects for things such as boosting, the crowd, and also noises for clicking scrolling and going back on buttons. This greatly helped to make the menus feel far more satisfying and responsive to player input.
The last thing I did was finally smooth out loading between Unity scenes. It used to just hard cut essentially to the next scene as it loaded. I have changed this so that when a new scene is loaded it fades to black and then out as well as the audio. This makes the game more polished to play and helps hide abrupt jumps in loading. I do this through a sprite that I change the alpha off through a coroutine and have it to not destroy on load.
private void Awake()
{
DontDestroyOnLoad(gameObject);
Color color = transitionImage.color;
color.a = 0f;
transitionImage.color = color;
transitionImage.gameObject.SetActive(false);
}
In the Awake Method I set it as don't destroy on load. I then turn it off as I dont want it to interfere with my UI and not being able to press buttons.
private IEnumerator FadeOutAndLoadCoroutine(string sceneName)
{
transitionImage.gameObject.SetActive(true);
yield return StartCoroutine(Fade(0f, 1f));
// load the next scene
yield return SceneManager.LoadSceneAsync(sceneName);
yield return null;
yield return StartCoroutine(FadeInCoroutine());
}
This method shows that I initiate fade to black (1f alpha value), load the scene, wait a frame, and then start the fading back to clear coroutine.
The fade itself is also done through code. I handled it this way so I can manually change how long the 'loading' screen is.
private IEnumerator Fade(float startAlpha, float endAlpha)
{
float elapsedTime = 0f;
Color color = transitionImage.color;
while (elapsedTime < fadeDuration)
{
elapsedTime += Time.deltaTime;
color.a = Mathf.Lerp(startAlpha, endAlpha, elapsedTime / fadeDuration);
transitionImage.color = color;
yield return null;
}
color.a = endAlpha;
transitionImage.color = color;
}
I then reference this SceneTransition script in my game manager so that when the player presses a button to go to a different scene the fade is triggered.
You can see this all in action here:
Comments